By default emacs has line wrap enabled for the whole window. I.e if you only have 1 buffer open then it will line wrap at the edge of the window.

If you open many buffers though there is no line wrapping on each buffer.
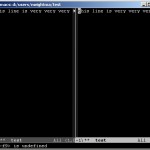
If you add the following to your .emacs file it will make side by side buffers behave like the full window.
(setq truncate-partial-width-windows nil)

I dont like line wrap, especially when coding so I set the following
(setq default-truncate-lines t)

I then use f12 to toggle the line wrap by adding the following
(global-set-key [f12] 'toggle-truncate-lines)
So my whole line-wrap.el file is
;; See http://www.delorie.com/gnu/docs/elisp-manual-21/elisp_620.html
;; and http://www.gnu.org/software/emacs/manual/elisp.pdf
;; disable line wrap
(setq default-truncate-lines t)
;; make side by side buffers function the same as the main window
(setq truncate-partial-width-windows nil)
;; Add F12 to toggle line wrap
(global-set-key [f12] 'toggle-truncate-lines)
See 38.3 Truncation or the emacs manual for more information.